Introduction
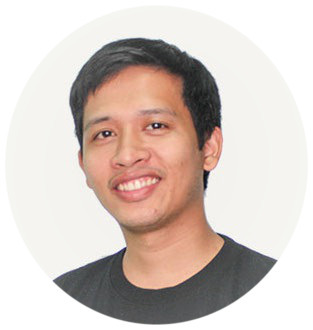
My name is Earl David Tianco of COMMUDE Philippines,
I started using Unity3D when I moved to Game Development for more than 6 years back, and I have made multiple applications and games thru the years. Now I am working with mobile applications, and Unity3D has never failed me. Until now, I have been continuously learning more features and processes as more projects come along, it continues to help me as a developer grow together with the company.
While anybody can learn Unity3D, not everyone knows the best practices in coding in Unity3D. We will be tackling some of the common best practices in Unity3D.
Introduction
In this vastly improving modern day and age, a lot of our world’s problems can be solved with technology, and yet there are still a lot of things remains unsolved. In software development, software engineers are earnestly looking for different ways to improve people’s lives. And for them to do this, they need help from different software tools. The tools I’m referring to are the programming languages, IDE (integrated development environment), engines, platform tools, or any software program they are comfortable to use. Unity3D is one of them.
Unity3D is a multi-platform game engine that was initially designed to help game developers develop 2D and 3D games, however because of it’s flexibility, user-friendliness, and a wide range of assets and plugins, it has also been used for different kind of business solutions, and other applications like Virtual Reality and Augmented Reality.
Software Design Patterns
In coding, it is very common to make use of software design patterns to help ease with programming functionalities. Having the whole team follow a certain design pattern will help with code readability while also improving your development processes, and this includes testing and debugging.
In Unity, one of the most commonly used is Singleton because of it’s convenience. It is mostly used when the project is required to use Managers such as GameManager, or GUIManager, and the like. With this, it is easier to access functions inside the Managers, and vice versa. Below is an example of a singleton software design pattern:
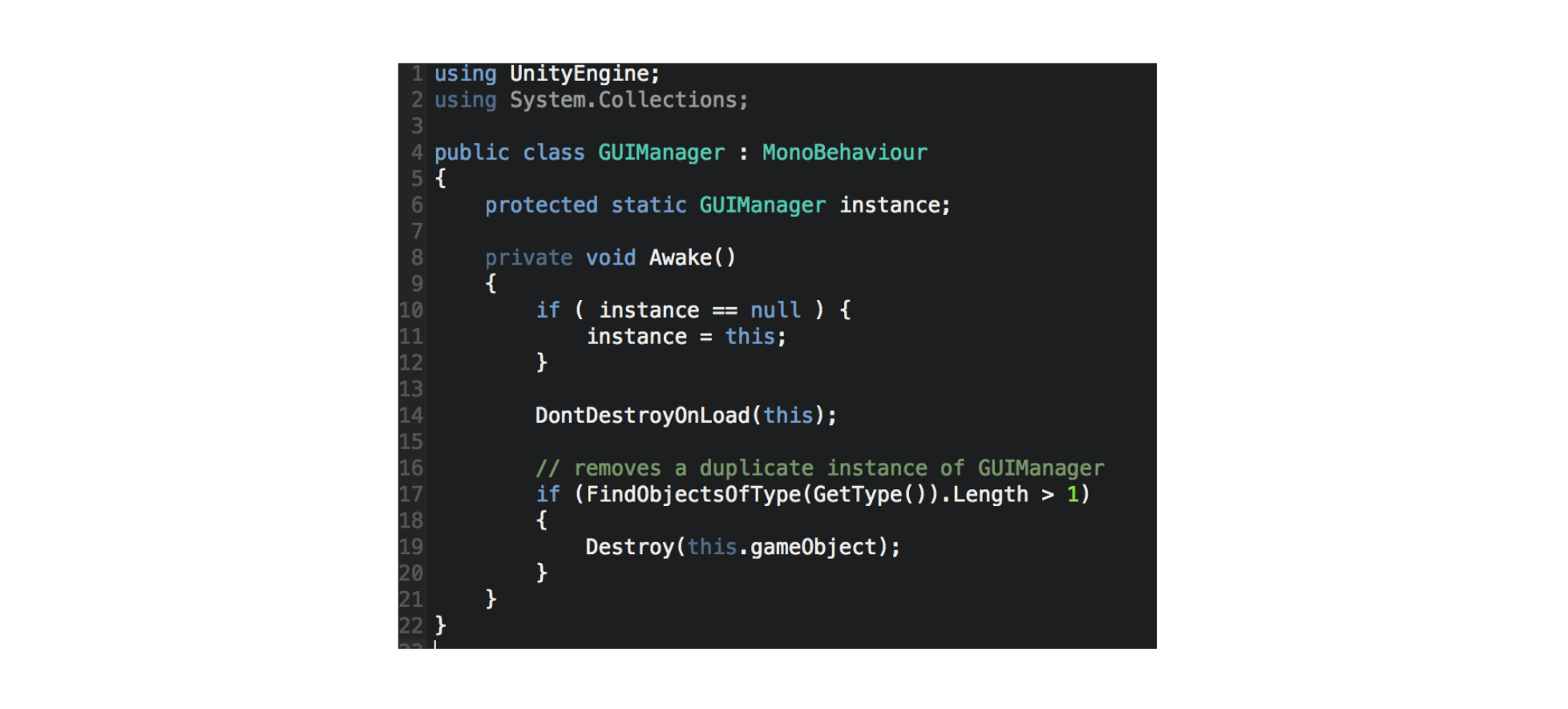
In creating Manager scripts, if you need to have a variable that is intended to be used by other scripts, it is better to use public variables as references other than using GameObject.Find() to locate for GameObjects. This will reduce the time it takes to run the script since it doesn’t need to locate the required variables as it is already referenced on the editor. However, if you only need the variable to be referenced, but not be used in any way publicly outside of it’s class then, you can create a private variable instead and have it set it’s attribute as a [SerializeField]. When adding [SerializeField] Attribute to a private variable, it will show itself to the editor, while still restricting it’s access with those outside of it’s script.
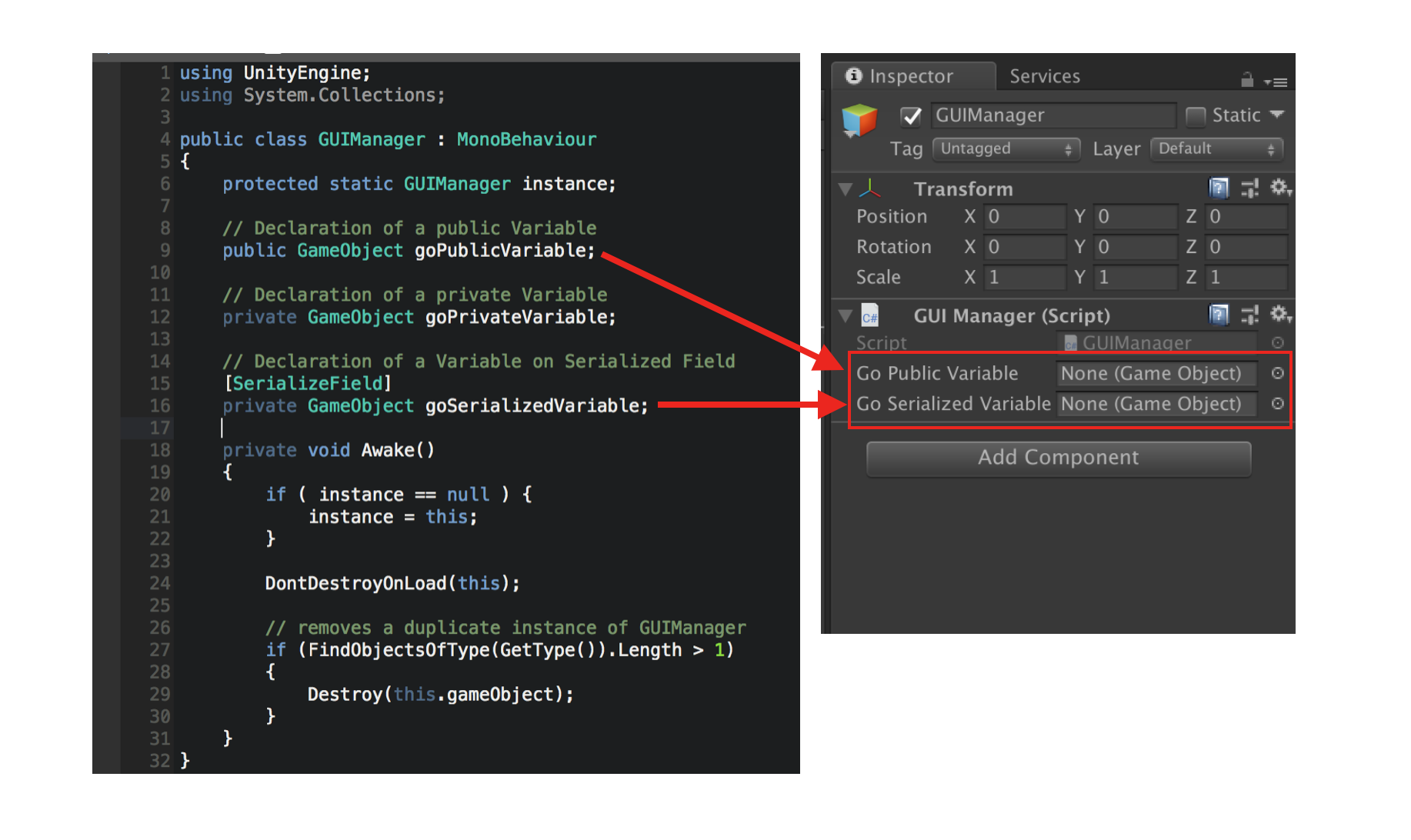
Notice that when a public variable is declared, it will be shown in the inspector, while if a variable is private, it doesn’t. However, if the private variable’s attribute is set as a [SerializeField], it will appear in the inspector similar to a public variable.
Naming Conventions
In software development, especially in Unity3D who uses C# or Javascript as it’s programming language, there are a set of guidelines and naming conventions that helps improve your coding quality, readability, and maintenance. This will not only be of great help to you especially on debugging and testing, but also will be easier for all of your team members to figure out the functionalities of the methods and easier to understand your code.
Always be careful when creating the names of variables, methods, classes, tags and functions as everything is case sensitive. For most of the time, it is a standard to use Upper Camel Case or “Pascal Case” on Class names (class SampleClass()), while Lower Camel Case on variable names (string sampleVariable), similar to the examples above. It is also a standard to create the names of the assets in your project in Pascal Case as well.
When creating functions or methods, it is a standard to have it’s name be descriptive understandable. So, in creating a function when pressing a button, instead of having a function named “Pressed()”, it is better to name it ButtonPressed() so that it is easier to understand that the function is called when the button is pressed.
It is a good coding practice to always place comments certain actions, functions or even variables to have other developers or team members know it’s functionality, or procedures. This is also a form of documentation so that your code is understandable even with those who are not really a developer. The more clearer your comments are, the better. Comments are those what you write after the double slash (//) or if you have multiple-lined comments, you can have them placed inside a block starting with slash-asterisk (/*) and ends with asterisk-slash (*/).
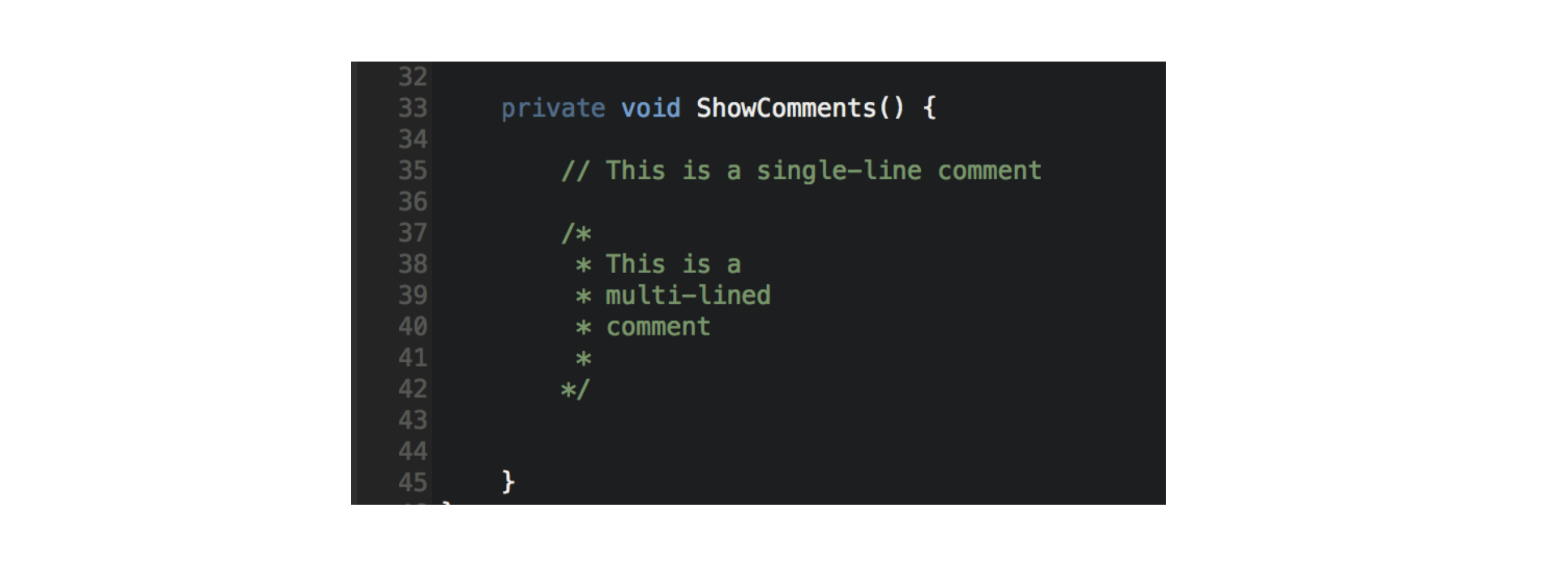
Prefabs are your friend
Always use prefabs! Prefabs are like a clone of a certain object in your project, regardless of what kind of object. It copies all of your original object and creates an instance of that object together will all it’s components. Therefore, you can actually make use of it as a template, and change it anything you like, without the worry of having to change your original object. Just think of it as similar to Version Control but for GameObjects.
Optimization
1. Before building the project, remember to remove all Debug functions you have placed inside your codes.
2. Don’t use repeating codes. If you see a block of code, with exactly the same block of code in another area, try and have it made into a method or function instead.
3. If you can break down one large function into small, concise ones to refrain from running unnecessary codes, then do it. The less work your code has to run, the better.
4. Instead of using GameObject.Find, it’s better to use references using public variables or SerializeField variables (preferably SerializeField).
5. Even though foreach() loop has advantages when looping into children of a GameObject, or Transform, it has a tendency to have memory leaks, so instead, it’s better to use for-loop as much as possible. Please note that even though it is better to use for-loop than foreach, it doesn’t mean that it is okay to use for loop or any other loop always.. it is still better to reduce the chance of running into loops to improve performance.
6. Make use of Unity’s Occlusion Culling, because this disables the rendering of objects outside of the camera or if they are behind other objects to have a better performance.
7. Always use the Unity’s Profiler. It is a great feature of Unity where it’s main purpose is to help you optimize your Project. It reports details of your project whether it is on Run-time or not, and you can analyze the performance the GPU, CPU, memory, rendering, audio, etc.
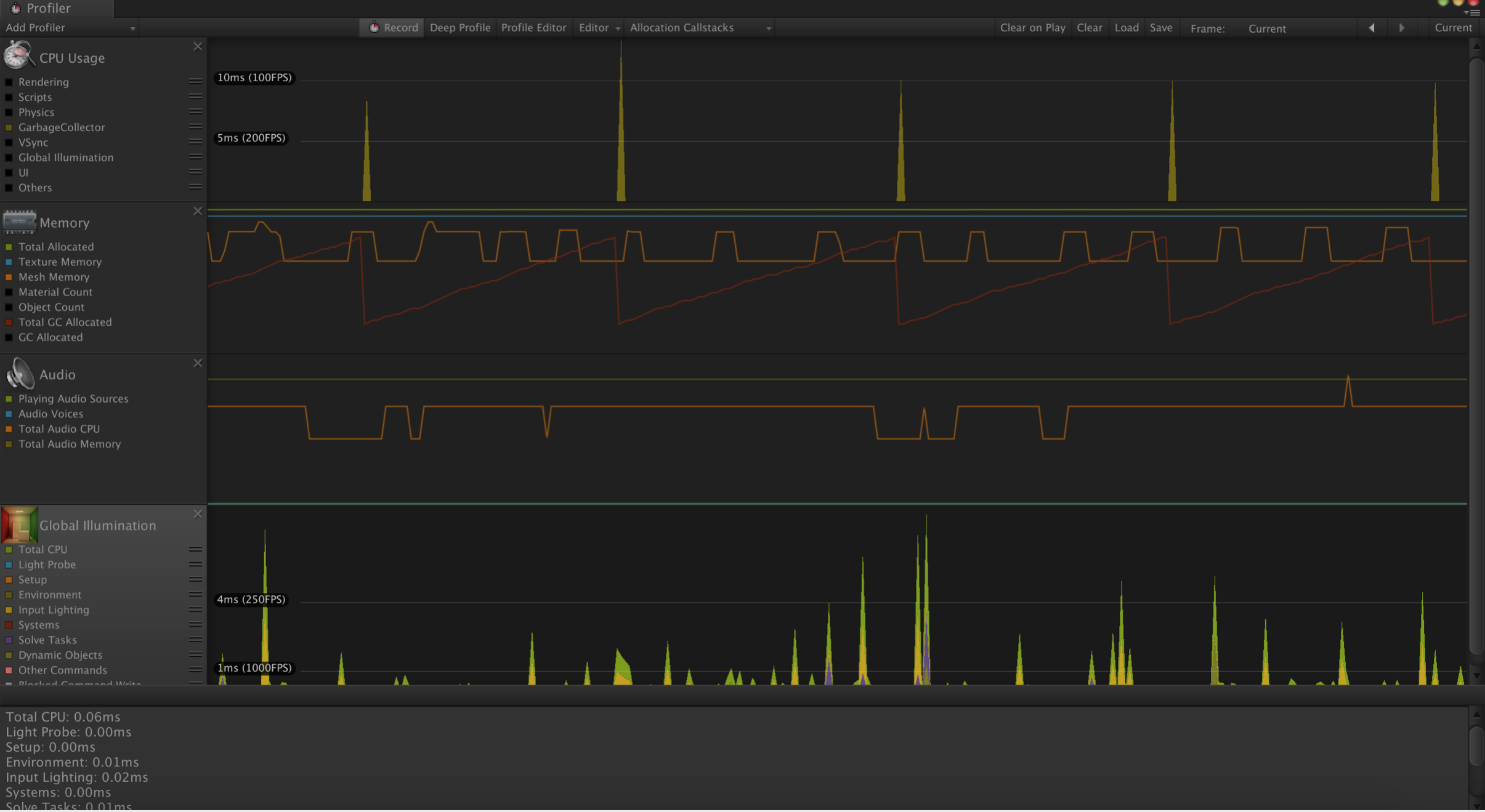
Unity Profiler
Version Control
“Version control, also known as revision control or source control, is the management of changes to documents, computer programs, large web sites, and other collections of information.”
In a project, it is usually required to have version control to track every changes made especially when handled by multiple developers. Most of the time, there will be instances when the scripts, scenes or assets you have created, updated or deleted will affect your team members especially when there are dependencies, therefore jeopardizing the whole project with a single mistake. The best way to avoid these kind of problems are using version control. This way, it will minimize if not remove problems regarding project management and development. It is also a good practice to create a clone or a clean copy of a project or at least have it updated, debugged and tested well before merging with the main copy of your project.
Conclusion
While Unity3D is one of the most used game engine as it has a lot to offer while being very user-friendly and flexible, this also means that it is also easy to make mistakes, or go into some trouble with performance issues, or team issues. While Unity3D’s community is very large, which is why it is also easy to ask for help in when issues arise, I think we should all understand the best practices and have our own coding standards so that we would have fewer mistakes, and improve our own coding performance, not only for ourselves but for the team as well.. so that one day we will be better software engineers than we are now.
References
https://docs.unity3d.com/Manual/Profiler.html
https://en.wikipedia.org/wiki/Version_control